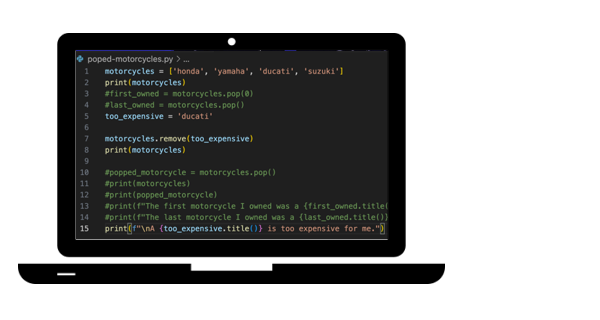
The fundamentals of Python:
1. Variables and Data Types
- Variables: Containers for storing data values. Example:
x = 5
- Data Types: Common types include integers (
int
), floating-point numbers (float
), strings (str
), lists (list
), tuples (tuple
), dictionaries (dict
), and boolean (bool
).
2. Operators
- Arithmetic Operators:
+
(addition),-
(subtraction),*
(multiplication),/
(division),%
(modulus),**
(exponentiation),//
(floor division). - Comparison Operators:
==
(equal),!=
(not equal),>
,<
,>=
,<=
. - Logical Operators:
and
,or
,not
.
3. Control Flow
- Conditional Statements:
if
,elif
,else
. Used to execute code based on conditions.pythonCopy codeif x > 0: print("Positive") elif x == 0: print("Zero") else: print("Negative")
- Loops:
for
andwhile
loops to repeat actions.pythonCopy codefor i in range(5): print(i) while x < 10: x += 1
4. Functions
- Defining Functions: Using the
def
keyword.pythonCopy codedef greet(name): return f"Hello, {name}!"
- Calling Functions: Executing the function with appropriate arguments. Example:
greet("Alice")
5. Data Structures
- Lists: Ordered and changeable collections. Example:
my_list = [1, 2, 3]
- Tuples: Ordered and unchangeable collections. Example:
my_tuple = (1, 2, 3)
- Dictionaries: Unordered collections of key-value pairs. Example:
my_dict = {"name": "Alice", "age": 25}
- Sets: Unordered collections of unique elements. Example:
my_set = {1, 2, 3}
6. String Manipulation
- Basic string operations like concatenation, slicing, and formatting.pythonCopy code
s = "Hello" print(s + " World") # Concatenation print(s[1:4]) # Slicing print(f"{s} World") # Formatting
7. File I/O
- Reading from and writing to files.pythonCopy code
with open('file.txt', 'r') as file: content = file.read() with open('file.txt', 'w') as file: file.write("Hello, World!")
8. Error Handling
- Using
try
,except
,else
, andfinally
to handle exceptions.pythonCopy codetry: x = 1 / 0 except ZeroDivisionError: print("Cannot divide by zero")
9. Modules and Packages
- Importing and using external libraries.pythonCopy code
import math print(math.sqrt(16))
10. Basic Object-Oriented Programming (OOP) Concepts
- Classes and Objects: Creating and using classes and objects.pythonCopy code
class Dog: def __init__(self, name): self.name = name def bark(self): print("Woof!") my_dog = Dog("Rex") my_dog.bark()
These fundamentals provide a solid foundation for beginners to start coding in Python. As they progress, they can delve deeper into more advanced topics and libraries.